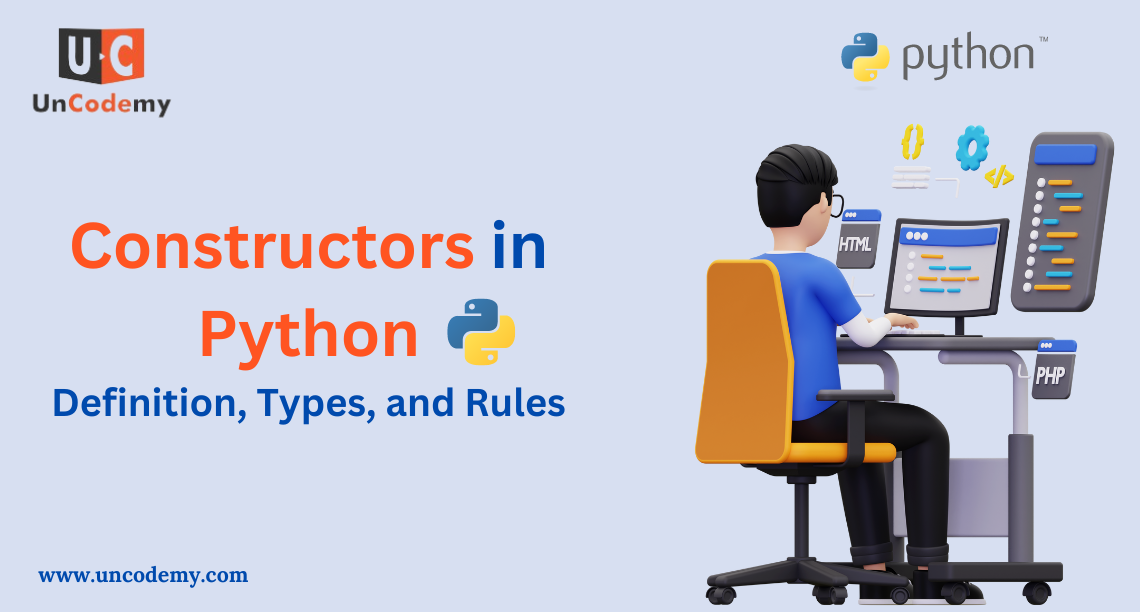
Constructors in Python are an essential part of object-oriented programming (OOP). In Python, constructors are special methods that are used to initialize the attributes of a class and they are called automatically when an object of a class is created. In this article, we will discuss constructors in Python, including their definitions, types, and rules.
What is a Constructor in Python?
In Python, the constructor is defined using the init() method. The method’s name must be init(), and it must take at least one argument, which is usually self.
Types of Constructors in Python
There are two types of constructors in Python:
- Default Constructor
- Parameterized Constructor
Default Constructor
A default constructor in Python is a constructor that takes no arguments. It is created automatically if no constructor is defined for a class. It initializes the attributes of a class with default values. For example, if we define a class without a constructor, a default constructor will be created automatically:
class MyClass: pass
The above code defines a class called MyClass without a constructor. A default constructor will be created automatically. If we create an object of MyClass, the default constructor will be called:
obj = MyClass()
Parameterized Constructor
A parameterized constructor is a constructor that takes one or more arguments and is used to initialize the attributes of a class with user-defined values. For example, let’s define a class with a parameterized constructor:
class MyparameterisedConst: def __init__(self, arg1, arg2): self.attribute1 = arg1 self.attribute2 = arg2
The above code defines a class called MyparameterisedConst with a parameterized constructor. The constructor takes two arguments, arg1, and arg2, and initializes the attributes attribute1 and attribute2 with these values. If we create an object of MyparameterisedConst with arguments, the parameterized constructor will be called:
obj = MyparameterisedConst(“value1”, “value2”)
In the above code, we create an object of MyparameterisedConst with arguments “value1” and “value2”. The parameterized constructor will be called, and the attributes attribute1 and attribute2 will be initialized with these values.
Rules for Constructors in Python
Some rules that you will learn in the Python training course and that must be followed while defining constructors in Python:
- init() must be the name of the constructor.
- The constructor must take at least one argument, which is usually self.
- The constructor can take any number of arguments, but the first argument must always be self.
- The constructor can have default values for its arguments.
- The constructor can be overloaded, meaning multiple constructors can be defined for a class with different numbers and types of arguments.
Let’s discuss these rules in more detail:
1 – init() must be the name of the constructor:
In Python, init() is a special method that is called automatically when an object of the class is created. If the constructor is named anything other than init(), it will not be called automatically.
2 – The constructor must take at least one argument, which is usually self:
The constructor must take at least one argument, which is usually self. The self-argument refers to the object itself and is used to access the attributes and methods of the object. Without the self-argument, the constructor will not be able to access the attributes and methods of the object.
3 – The constructor can take any number of arguments, but the first argument must always be self:
The constructor can take any number of arguments, but the first argument must always be self. This is because the self-argument refers to the object itself, and it must always be the first argument in any method of a class.
The constructor can have default values for its arguments.
The constructor can have default values for its arguments. This means that if an argument is not passed when creating an object of the class, the default value will be used instead. For example:
class MyClass:
def init(self, arg1=”default_value”):
self.attribute1 = arg1
In the above code, the parameterized constructor takes one argument, arg1, which has a default value of “default_value”. If we create an object of MyClass without passing any arguments, the default value will be used:
obj = MyClass()
print(obj.attribute1) # Output: “default_value”
4 – The constructor can be overloaded
The constructor can be overloaded, meaning multiple constructors can be defined for a class with different numbers and types of arguments. This allows us to create objects of a class with different sets of attributes. To define multiple constructors for a class, we can use the @staticmethod or @classmethod decorators.
What are Static Methods?
Static methods are methods that do not require access to the object or its attributes. They are defined using the @staticmethod decorator. To define a static method as a constructor, we can use the following syntax:
class MyClass:
def init(self, arg1, arg2):
self.attribute1 = arg1
self.attribute2 = arg2
@staticmethod def from_list(list): return MyClass(lst[0], lst[1])
In the above code, we define a parameterized constructor that takes two arguments, arg1 and arg2. We also define a static method called from_list() that takes a list as an argument and returns an object of MyClass with the first two elements of the list as its attributes. This allows us to create objects of MyClass with different sets of attributes:
obj1 = MyClass(“value1”, “value2”)
obj2 = MyClass.from_list([“value3”, “value4”])
print(obj1.attribute1, obj1.attribute2) # Output: “value1 value2”
print(obj2.attribute1, obj2.attribute2) # Output: “value3 value4”
What are class methods?
Class methods are methods that require access to the class itself rather than the object. They are defined using the @classmethod decorator. To define a class method as a constructor, we can use the following syntax:
class MyClass:
def init(self, arg1, arg2):
self.attribute1 = arg1
self.attribute2 = arg2
@classmethod def from_dict(cls, dct): return cls(dct[“key1”], dct[“key2”])
In the above code, we define a parameterized constructor that takes two arguments, arg1, and arg2. We also define a class method called from_dict() that takes a dictionary as an argument and returns an object of MyClass with the values of the keys “key1” and “key2” as its attributes. This allows us to create objects of MyClass with different sets of attributes:
obj1 = MyClass(“value1”, “value2”)
obj2 = MyClass.from_dict({“key1”: “value3”, “key2”: “value4”})
print(obj1.attribute1, obj1.attribute2) # Output: “value1 value2”
print(obj2.attribute1, obj2.attribute2) # Output: “value3 value4”
Conclusion
Constructors are an essential part of object-oriented programming in Python. In the Python training course, you will learn that they are used to initialize the attributes of objects when they are created. The constructor method is called automatically when an object is created using the class name followed by parentheses. The self-argument refers to the object itself, and it must always be the first argument in any method of a class.
In Python, constructors can have default values for their arguments, which are used if an argument is not passed when creating an object of the class. Additionally, constructors can be overloaded, meaning multiple constructors can be defined for a class with different numbers and types of arguments. This allows us to create objects of a class with different sets of attributes.
Static methods and class methods can also be used as constructors by using the @staticmethod and @classmethod decorators, respectively. Static methods do not require access to the object or its attributes, while class methods require access to the class itself.
Overall, constructors are an important part of object-oriented programming in Python, and understanding how to use them effectively can make your code more efficient and flexible.